Introduction
At the beginning of 2016, when I was playing with Lambdas while working on my own projects, I had a script written in Nodejs which took care of deployment. It was a simple piece of code which zipped the source code, and with the help of AWS SDK, then created or deleted individual lambda functions.
After some time, at the end of the year 2017, when I was already working at Purple Technology and we had decided to go for Serverless, we researched what type of Serverless tooling was available. The best choice for us was the Serverless Framework, which was quite sophisticated even back then, with various plugins available, such as the “serveless-step-functions”—plugin which was and still is essential to us.
As I have already mentioned in the previous article, here in Purple Technology we only use AWS as a serverless provider, which means this article will be primarily focused on the Serverless Framework for AWS.
Serverless Framework Introduction
The Serverless Framework is a CLI tool that provides the deployment of infrastructure required for your serverless application to run seamlessly. The installation is performed through NPM and the configuration is done via a “serverless.yml” file. It supports around 10 cloud providers, such as AWS, Azure, and GCP and already has a fairly extensive plugin ecosystem—thanks to which it can be adjusted to suit your needs.
In the case of AWS, the Serverless Framework uses to manage the infrastructure IaC service called AWS CloudFormation. The Serverless Framework can generate a CloudFormation template for the necessary resources itself, however, if you want to configure other custom resources, e.g., DynamoDB tables, due to its openness, you can do it.
Installation and environment setup
Serverless Framework
$ npm install -g serverless
AWS CLI
Although the installation of the AWS CLI is not necessary, it will make the initial setup easier.
Documentation on how to install the AWS CLI can be found in the following link:
https://docs.aws.amazon.com/cli/latest/userguide/install-cliv2.html
AWS CLI Setting
$ aws configure
In this step, the most important thing will be filling in the AWS Access Key ID
and the AWS Secret Access Key
.
On the contrary, values such as Default region name
or Default output format
can be left blank or you can use their default values.
The AWS CLI creates the files ~/.aws/credentials
and ~/.aws/config
in this step, from which it implicitly takes access data for both the AWS CLI and all of the AWS SDK.
Documentation on how to get access credentials to the AWS API can be found here:
https://docs.aws.amazon.com/general/latest/gr/aws-sec-cred-types.html#access-keys-and-secret-access-keys
Creating a project according to a template
The Serverless Framework offers the possibility to create a new project using a template. In our case we will look for the templates with the aws-
prefix.
After template generation, lots of explanatory comments are in the serverless.yml
. After deleting and cleaning them, the serverless file looks like this:
service: two-serverless-framework
provider:
name: aws
runtime: nodejs12.x
region: eu-central-1
functions:
hello:
handler: handler.hello
Next, the Serverless Framework creates a handler.js
file:
'use strict';
module.exports.hello = async event => {
return {
statusCode: 200,
body: JSON.stringify(
{
message: 'Go Serverless v1.0! Your function executed successfully!',
input: event,
},
null,
2
),
};
};
The most important connection between the serverless.yml
and the handler.js
files is the handler: handler.hello
and module.exports.hello
. For a Node.js Lambda function, the path to the file always has to be specified, and, instead of a suffix, the name of the exported function needs to be stated. So, in this case the file path is the path to the handler
and the name of the exported function is hello
, which means that we insert handler.hello
into our serverless.yml
. For example, if the handler
file was in the src/
folder, the value in the serverless.yml
would be src/handler.hello
.
Node.js runtime supports two ways to return responses:
- Outdated – using callback
- Classic – using Promise
You can find more on the following page: https://docs.aws.amazon.com/lambda/latest/dg/nodejs-handler.html
The generated function “hello” is ready to be used along with an HTTP event. Nevertheless, we will take a look at that in the upcoming part, which will be dedicated to Amazon API Gateway.
Tip: list of AWS regions:
https://docs.aws.amazon.com/general/latest/gr/rande.html
Deployment
$ serverless deploy
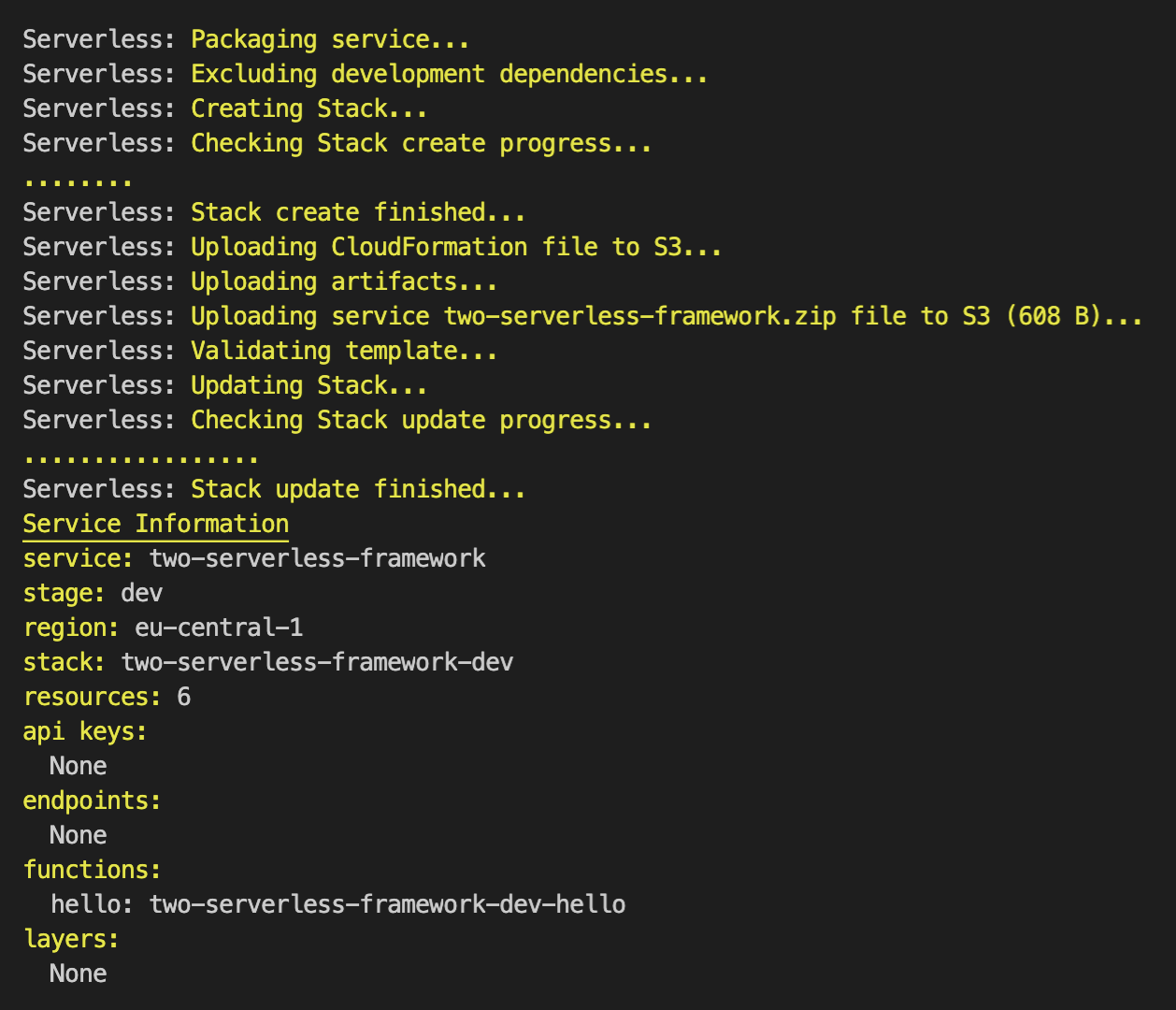
Once deployment is finished, we can see that the function hello was created and its name in AWS is two-serverless-framework-dev-hello
. So, if we look at the AWS console and enter this name into the search, we get our Lambda function.
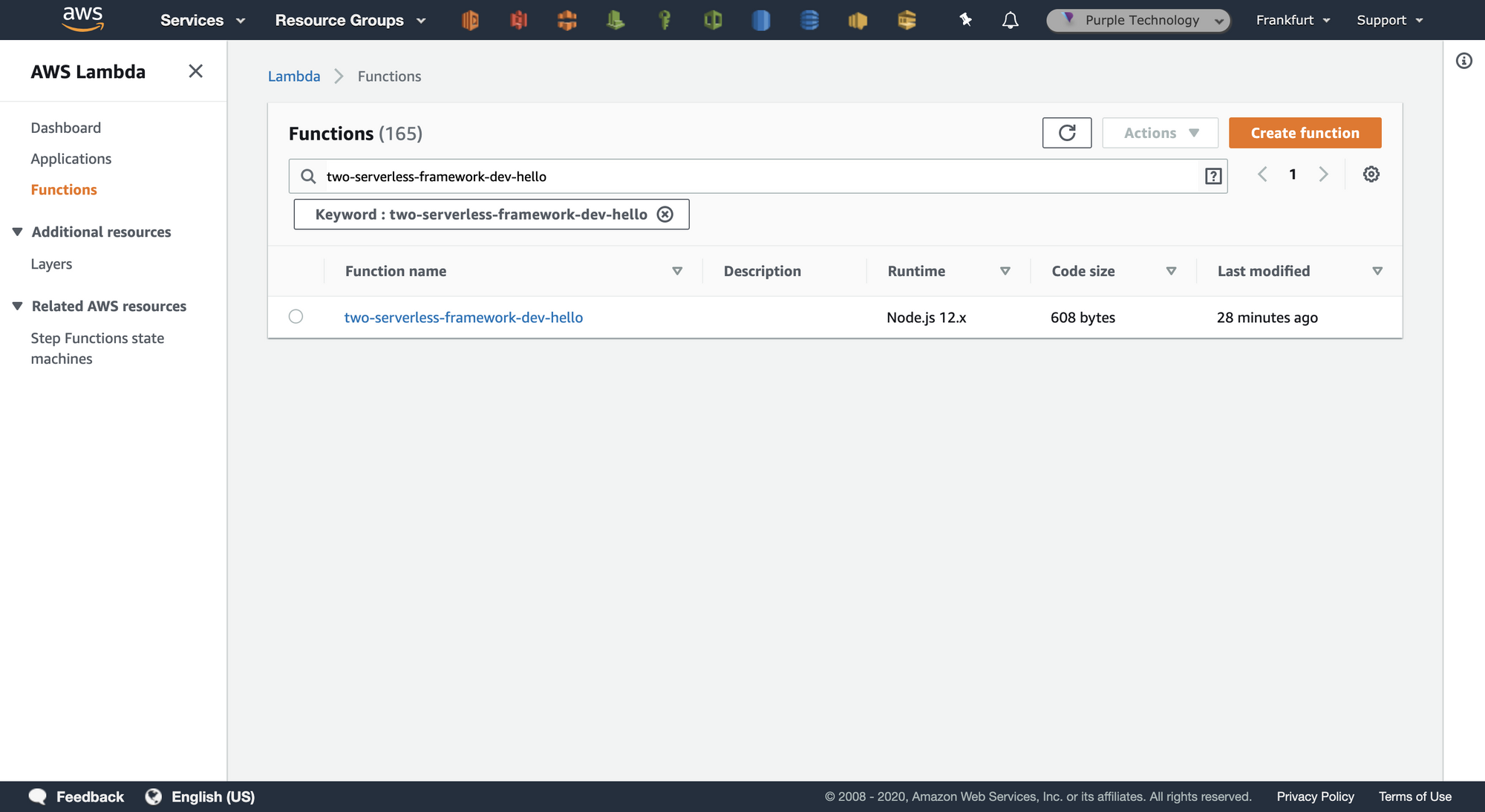
Tip: In case we want to display this information for an already deployed application, we can do so by using the commandserverless info
, or for more informationserverless info -v
Removing
$ serverless remove
Removing a deployment usually consists of deleting the S3 bucket content which contains the zipped source code of the lambda functions, followed by deleting the CloudFormation stack.
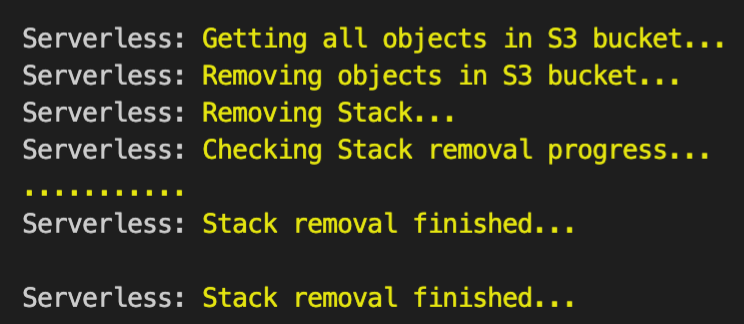
AWS CloudFormation
As I have already mentioned in the introduction, the Serverless Framework for AWS uses the AWS Cloud Formation service; it means that the Serverless Framework only starts the deployment and removal process, and the deployment/removal process itself is performed by AWS CloudFormation.
It means that in the case of deployment or removal of a stack, when the Serverless Framework shows only dots like in the following example Serverless: Checking Stack removal progress...........
and you close the terminal, nothing will happen as the process in CloudFormation has already started. The only thing the Serverless Framework is doing at that moment is continually asking the AWS API if it has finished, so it knows when to end.
CloudFormation is a complex and powerful tool, and I will elaborate on that more in a separate article.
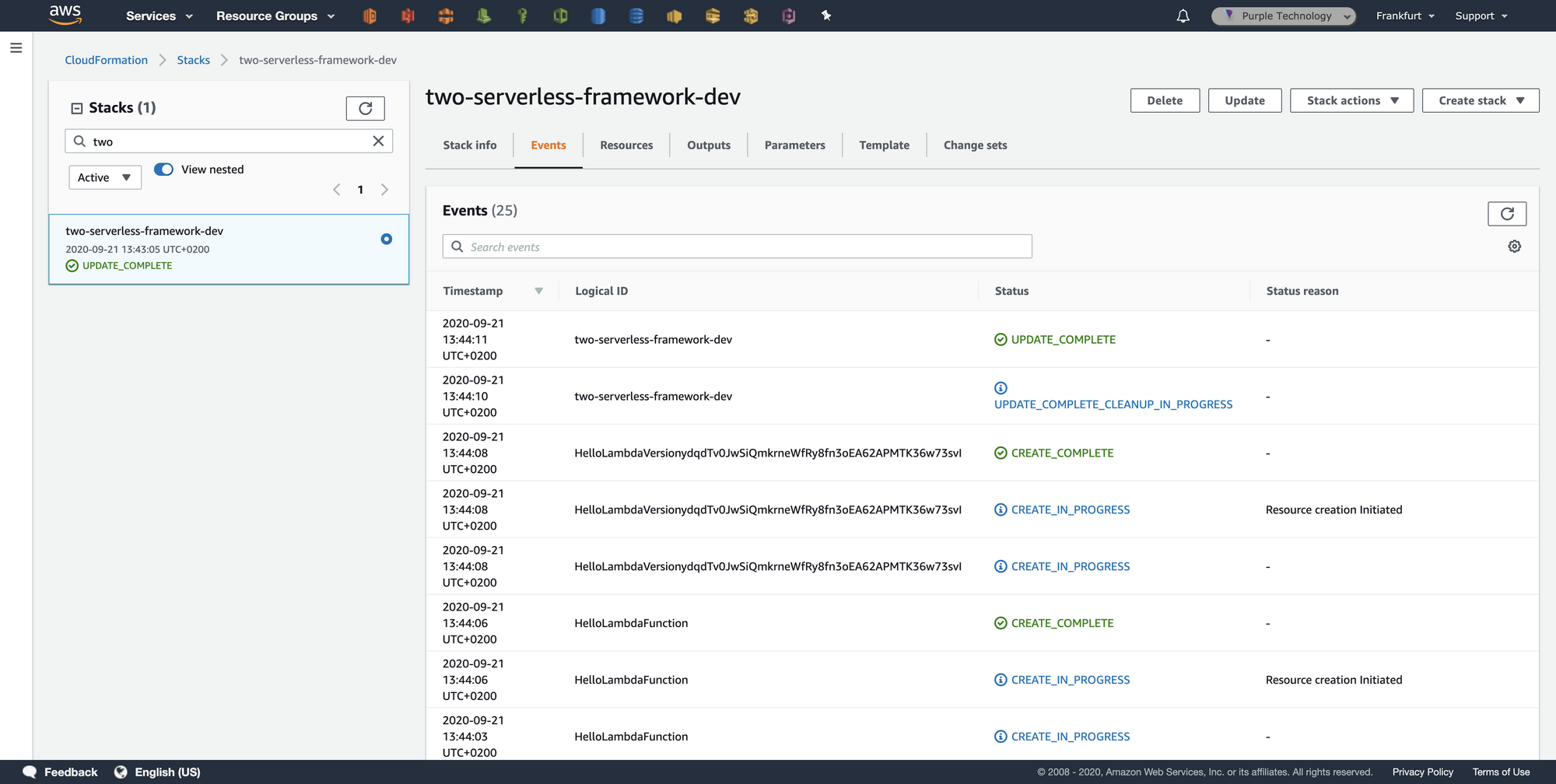
Plugins
The Serverless Framework supports the possibility to extend its basic functionality by using plugins. Unfortunately, there is basically no documentation on how to create and write plugins, and therefore you have to explore the Serverless Framework yourself. We in Purple Technology have written a few plugins for our internal use and this might be a topic for a future article.
Plugins which are used in Purple Technology:
- serverless-prune-plugin – A plugin which helps avoid running into the “Function and layer storage“ limit
- serverless-step-functions – Enables the deployment of State Machines
- serverless-plugin-aws-alerts – Allows for the monitoring of errors in various functions via AWS Chatbot
- serverless-webpack – enables us to use TypeScript for the Lambda functions instead of Javascript
- serverless-finch – Loads static files for the frontend into an S3 bucket during deployment
- serverless-s3-remover – Removes all files from the fronted S3 bucket before deletion of a CloudFormation stack
- @purple/serverless-git-branch-stage-plugin - Translates git branch name to Serverless Framework stage friendly form
Conclusion
In the following parts of this series, I’ll focus on the practical usage of the Serverless Framework, combined with different kinds of AWS services, e.g. API Gateway, DynamoDB, AppSync, S3, etc.
In case you have any questions, feel free to contact me at Twitter @FilipPyrek.
With ❤️ made in Brno.